particle Package¶
The particle package provides classes which represent OTT particles.
Particle
instances combine the optical scattering method and
drag calculation method into a single representation. There are currently
two specialisations of Particle
, summarised in
Fig. 6: Fixed
stores
the geometry, T-matrix and drag for a particle; Variable
re-calculates the T-matrix and drag whenever the refractive index of
particle geometry changes.
Unlike ott.tmatrix.Tmatrix
instances, Particle
instances
use SI units (i.e., the particle geometry and position uses units of meters).
In a future version this interface may change to support other scattering methods, and/or more optimal T-matrix calculation methods (such as only building required columns of the T-matrix depending on the incident beam).
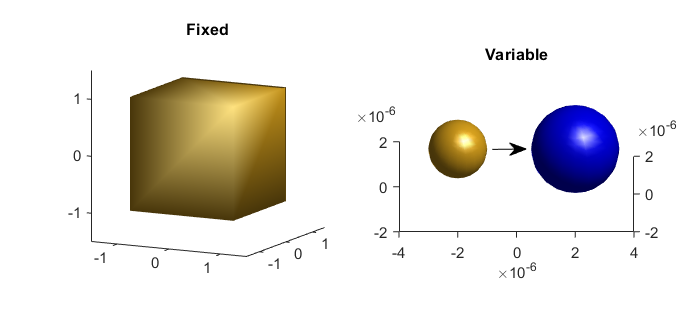
Fig. 6 Graphical display of the two different particle representations: fixed, which represents a constant particle; and variable, which represents a particle whose properties dynamicaly change.¶
Particle¶
- class ott.particle.Particle¶
Base class for particles in optical tweezers simulations. Inherits from
ott.utils.RotationPositionProp
and matlab.mixin.Hetrogeneous.This class combined the optical scattering methods, drag calculation methods and other properties required to simulate the dynamics of a particle in an optical tweezers simulation. In future version of OTT, this class may change to support multiple scattering methods.
This is an abstract class. For instances of this class see
Variable
and :class:Fixed`.- Abstract properties
shape – Geometric shape representing the particle
tmatrix – Describes the scattering (particle-beam interaction)
drag – Describes the drag (particle-fluid interaction)
tinternal – T-matrix for internal scattered field (optional)
mass – Particle mass [kg] (optional)
moment – Particle moment of inertia [kg m^2] (optional)
- Properties
position – Particle position [m]
rotation – Particle orientation (3x3 rotation matrix)
- Methods
surf – Uses the shape surf method to visualise the particle
setMassFromDensity – Calcualte mass from homogeneous density
- surf(particle, varargin)¶
Generate visualisation of the shape using the shape.surf method.
Applies the particle rotation and translation to the shape before visualisation.
- Usage
[…] = particle.surf(…)
For full details and usage, see
ott.shape.Shape.surf()
.
Fixed¶
- class ott.particle.Fixed(varargin)¶
A particle with stored drag/tmatrix properties. Inherits from
ott.particle.Particle
.- Properties
drag – Description of drag properties
tmatrix – Description of optical scattering properties
shape – Description of the geometry [m]
tinternal – Internal T-matrix (optional)
- Static methods
FromShape – Construct a particle from a shape description
- Fixed(varargin)¶
Construct a new particle instance with fixed properties
- Usage
particle = Fixed(shape, drag, tmatrix, …)
- Optional named arguments
shape (ott.shape.Shape) – Geometry description. Units: m. Default:
[]
.drag (ott.drag.Stokes) – Drag tensor description. Default:
[]
.tmatrix (ott.tmatrix.Tmatrix) – Scattering description. Default:
[]
.tinternal (ott.tmatrix.Tmatrix) – Internal T-matrix. Default:
[]
.mass (numeric) – Particle mass. Default:
[]
.
- static FromShape(shape, varargin)¶
Construct a particle from a shape description.
- Usage
particle = Fixed.FromShape(shape, …)
- Parameters
shape (ott.shape.Shape) – Particle geometry. [m]
- Named parameters
index_relative (numeric) – Relative refractive index of particle. Default:
[]
. Must be supplied or computable from index_particle and index_medium.index_particle (numeric) – Refractive index in particle. Default:
[]
.index_medium (numeric) – Refractive index in medium. Default:
1.0
unless both index_relative and index_particle are supplied. In which case index_medium is ignored.wavelength0 (numeric) – Vacuum wavelength. [m] This parameter is required to calculate the T-matrix.
viscosity (numeric) – Viscosity of medium. [Ns/m2] Default:
8.9e-4
(approximate viscosity of water).internal (logical) – If the internal T-matrix should also be computed. Default:
false
.mass (numeric) – Particle mass. Default:
[]
.
Variable¶
- class ott.particle.Variable(varargin)¶
A particle whose drag/tmatrix are automatically recomputed. Inherits from
ott.particle.Particle
.Changing the shape or refractive index of this particle causes the T-matrix and drag data to be re-calculated. This is useful for modelling particles with time-varying properties.
- Properties
shape – Shape describing the object (changes tmatrix/drag)
index_relative – Particle refractive index (changes tmatrix)
tmatrix_method – Method for T-matrix calculation
tinternal_method – Internal T-matrix calculation methdo
drag_method – Method for drag calculation
- Dependent properties
drag – Description of drag properties
tmatrix – Description of optical scattering properties
tinternal – Internal T-matrix (optional)
- Methods
setProperties – Set shape and index_relative simultaneously
- Static methods
FromShape – Create instance using FromShape of Tmatirx/Stokes
Sphere – Construct instance using Sphere approximation
StarShaped – Construct instance for star shaped particles
- static FromShape(varargin)¶
Construct a new Variable particle using the FromShape method.
This function sets up the
tmatrix_method
,tinternal_method
anddrag_method
functions to use theFromShape
methods fromott.tmatrix.Tmatrix
andott.drag.Stokes
.- Usage
particle = ott.particle.Variable.FromShape(…)
- Optional named arguments
wavelength_medium (numeric) – Medium wavelength. [m] Used for converting shape units [m] to wavelength units. Default:
1064e-9
(a common IR trapping wavelength).viscosity (numeric) – Viscosity of medium. [Ns/m2] Default:
8.9e-4
(approximate viscosity of water).internal (logical) – If the internal T-matrix calculation method should also be set. Default:
false
.
Unmatched parameters passed to class constructor.
- static Sphere(varargin)¶
Construct a variable particle for a spherical geometry
Uses the
FromShape
methods fromott.tmatrix.Mie
andott.drag.StokesSphere
.- Usage
particle = ott.particle.Variable.Sphere(…)
- Optional named arguments
wavelength_medium (numeric) – Medium wavelength. [m] Used for converting shape units [m] to wavelength units. Default:
1064e-9
(a common IR trapping wavelength).viscosity (numeric) – Viscosity of medium. [Ns/m2] Default:
8.9e-4
(approximate viscosity of water).internal (logical) – If the internal T-matrix calculation method should also be set. Default:
false
.
Unmatched parameters passed to class constructor.
- static StarShaped(varargin)¶
Construct a variable particle for a star shaped geometry.
Uses the
FromShape
methods fromott.tmatrix.Tmatrix
andott.drag.StokesStarShaped
.- Usage
particle = ott.particle.Variable.StarShaped(…)
- Optional named arguments
wavelength_medium (numeric) – Medium wavelength. [m] Used for converting shape units [m] to wavelength units. Default:
1064e-9
(a common IR trapping wavelength).viscosity (numeric) – Viscosity of medium. [Ns/m2] Default:
8.9e-4
(approximate viscosity of water).internal (logical) – If the internal T-matrix calculation method should also be set. Default:
false
.
Unmatched parameters passed to class constructor.
- Variable(varargin)¶
Construct a new Variable particle instance.
- Usage
particle = Variable(…)
- Optional named arguments
tmatrix_method ([] | function_handle) – T-matrix calculation method. Signature:
@(shape, ri, old_tmatrix)
. Default:[]
.drag_method ([] | function_handle) – Drag calculation method. Signature:
@(shape, old_drag)
. Default:[]
.tinternal_method ([] | function_handle) – internal T-matrix method. Signature:
@(shape, ri, old_tmatrix)
. Default:[]
.initial_shape ([] | ott.shape.Shape) – Initial particle geometry. Default:
[]
.initial_index_relative (numeric) – Initial particle relative refractive index. Default:
[]
.