beam Package¶
The beam package provides classes representing optical tweezers toolbox
beams. The base class for beams is Beam
. Sub-classes provide
beam specialisations for specific beam types (such as Gaussian
,
PlaneWave
, Webber
), point matching for modelling the
fields at the back aperture of a objective (PmParaxial
),
arrays of beams (Coherent
, Incoherent
), and
Scattered
beams. A summary is presented in
Fig. 5.
Unlike ott.bsc.Bsc
instances, Beam
instances
use SI units (i.e., force in Newtons, distance in meters).
Internally, the Beam
classes use ott.bsc.Bsc
to represent
the fields. Most class properties are defined in separate classes (declared
in the properties
sub-package.
In a future release of OTT, the Beam
interface may
change to support other types of field representations.
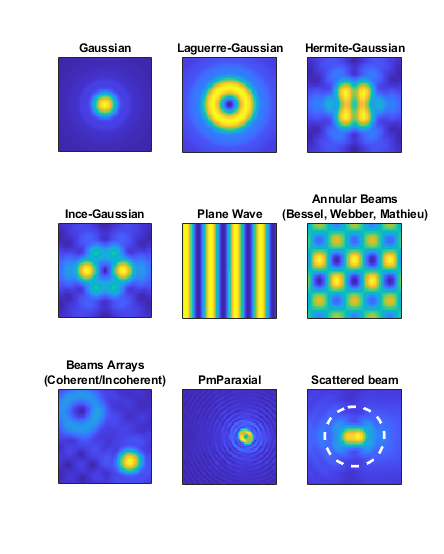
Fig. 5 Graphical display of the different kinds of beams currently included in the optical tweezers toolbox.¶
Contents
Base Classes¶
Beam¶
- class ott.beam.Beam¶
Provides a high-level view of a optical tweezers toolbox beam. Inherits from
ott.utils.RotationPositionProp
and matlab.mixin.Hetrogeneous.This class is the base class for OTT beams.
Beam
and its sub-classes provide a description of beams but do not implement any of the field or scattering calculation methods. These classes separate properties describing the beam (such as position, power and waist) from properties specific to the numerical calculation (VSWF data, Nmax, apparent power). Internally,Beam
usesott.bsc.Bsc
for field calculations. Depending on the implementation, theott.bsc.Bsc
data is either stored or calculated when required.The other major difference from
ott.bsc.Bsc
is the units.Beam
uses SI units for all quantities, making integration with dynamics simulations easer.In OTTv2, this interface will likely be extended to other types of beam/scattering methods (such as paraxial beams or other approximations).
- Properties
position – (3x1 numeric) Location of the beam
rotation – (3x3 numeric) Orientation of the beam
wavelength – Wavelength in medium [m]
wavenumber – Wavenumber in medium [1/m]
speed – Speed of light in the medium [m/s]
speed0 – Speed of light in vacuum [m/s]
scale – Scaling parameter for beam fields (default: 1)
- Abstract properties
omega – Optical angular frequency of light [1/s]
index_medium – Refractive index of the medium
- Methods
setWavelength – Set wavelength property
setWavenumber – Set wavenumber property
scatter – Calculate how a beam is scattered
- Field calculation methods
ehfield – Calculate electric and magnetic fields around the origin
ehfieldRtp – Calculate electric and magnetic fields around the origin
ehfarfield – Calculate electric and magnetic fields in the far-field
eparaxial – Calculate electric fields in the paraxial far-field
hparaxial – Calculate magnetic fields in the paraxial far-field
ehparaxial – Calculate electric and magnetic paraxial far-fields
- Force and torque related methods
forcetorque – Calculate the force and the torque between beams
intensityMoment – Calculate moment of beam intensity in far-field
force – Calculate the change in momentum between two beams
torque – Calculate change in angular momentum between beams
spin – Calculate change in spin momentum between beams
- Field visualisation methods
visNearfield – Generate a visualisation around the origin
visFarfield – Generate a visualisation at the far-field
visFarfieldSlice – Visualise the field on a angular slice
visFarfieldSphere – Visualise the filed on a sphere
- Mathematical operations
times,mtimes – Scalar multiplication of beam fields
rdivide,mrdivide – Scalar division of beam fields
uminus – Flip beam field intensity
plus, minus – Combine beams coherently
or – Combine beams incoherently
- Abstract methods
efield – Calculate electric field around the origin
hfield – Calculate magnetic field around the origin
efieldRtp – Calculate electric field around the origin (sph. coords.)
hfieldRtp – Calculate magnetic field around the origin (sph. coords.)
efarfield – Calculate electric fields in the far-field
hfarfield – Calculate magnetic fields in the far-field
scatterInternal – Called to calculate the scattered beam
Empty¶
- class ott.beam.Empty(varargin)¶
A beam with no fields. Inherits from
Beam
.This class represents empty space with no fields. This is useful for default parameters to functions or for unassigned elements of beam arrays (part of Hetrogeneous interface).
- Properties
position - (3x1 numeric) Position of the empty space.
rotation - (3x3 numeric) Orientation of the empty space.
index_medium – Refractive index of the medium
wavelength – Wavelength in medium [m]
wavenumber – Wavenumber in medium [1/m]
omega – Optical angular frequency of light [1/s]
speed – Speed of light in the medium [m/s]
speed0 – Speed of light in vacuum [m/s]
- Methods
efield, hfield, ehfield, … – Returns zeros
scatter – Returns a scattered beam with empty parts
BscBeam¶
- class ott.beam.BscBeam(varargin)¶
Beam class encapsulating a BSC instance. Inherits from
ott.beam.ArrayType
.This class stores an internal
ott.bsc.Bsc
instance which it uses for calculating fields.Methods in this class assume the beam is a regular beam (i.e., not the outgoing fields from a scattered beam).
- Properties
data – Internal BSC instance describing beam
apparentPower – Apparent power of the BSC data
- Methods
recalculate – Can be overloaded by sub-classes to update data
efield, hfield, … – Calculate fields in SI units, uses the
ott.bsc.Bsc
methods internally.force, torque, spin – Calculate force/torque/spin in SI units
- Supported casts
ott.bsc.Bsc – Get the BSC data after applying transformations
Additional properties/methods inherited from
Beam
.
BscFinite¶
- class ott.beam.BscFinite(varargin)¶
A beam represented by a finite VSWF expansion. Inherits from
BscBeam
.This class describes beams which can be represented using a finite VSWF expansion. The class stores a
Bsc
instance internally. BSC coefficients at any other location can be found by applying a translation to the beam data.Far-fields are calculated without applying a translation to the BSC data, instead the fields are calculated at the origin and phase shifted.
As with the
BscBeam
class, this class assumes a regular beam.- Properties
power – Power applied to the beam in
ott.bsc.bsc
.
- Supported casts
ott.bsc.Bsc – Get the BSC data after applying transformations
Additional properties/methods inherited from
BscBeam
.
BscInfinite¶
- class ott.beam.BscInfinite(varargin)¶
Describes a beam with infinite spatial extent. Inherits from
BscBeam
.This class is useful for describing plane waves, annular beams, and other beams with an infinite spatial extent. Values are only valid within the Nmax region, requesting values outside this region requires the beam to be re-calculated (or, for plane waves/annular beams, translated).
This class overloads the field calculation functions and requests a larger Nmax whenever points outside the valid range are requested. Far-field functions raise a warning that fields may not look as expected.
- Properties
Nmax – Nmax of the stored data
For methods/properties, see
BscBeam
.
Scattered¶
- class ott.beam.Scattered(varargin)¶
Describes the beam scattered from a particle. Inherits from
ott.beam.Beam
.When the internal field and the particle are supplied, the visualisation and field calculation method calculate either the external or internal fields depending on the requested points location. The visualisation methods also provide an option for showing the particle outline.
- Properties
incident – Beam data incident on the particle
scattered – Beam data scattered by the particle
outgoing – Outgoing modes radiation from the particle
incoming – Incoming modes scattered by the particle
internal – Internal beam data (optional)
particle – Particle responsible for scattering (optional)
- Field calculation methods
efield – Calculate electric field around the origin
hfield – Calculate magnetic field around the origin
ehfield – Calculate electric and magnetic fields around the origin
efieldRtp – Calculate electric field around the origin (sph. coords.)
hfieldRtp – Calculate magnetic field around the origin (sph. coords.)
ehfieldRtp – Calculate electric and magnetic fields around the origin
efarfield – Calculate electric fields in the far-field
hfarfield – Calculate magnetic fields in the far-field
ehfarfield – Calculate electric and magnetic fields in the far-field
eparaxial – Calculate electric fields in the paraxial far-field
hparaxial – Calculate magnetic fields in the paraxial far-field
ehparaxial – Calculate electric and magnetic paraxial far-fields
- Force and torque related methods
force – Calculate the change in momentum between two beams
torque – Calculate change in angular momentum between beams
spin – Calculate change in spin momentum between beams
forcetorque – Calculate the force and the torque between beams
- Field visualisation methods
visNearfield – Generate a visualisation around the origin
visFarfield – Generate a visualisation at the far-field
visFarfieldSlice – Visualise the field on a angular slice
visFarfieldSphere – Visualise the filed on a sphere
Beam types¶
Gaussian¶
- class ott.beam.Gaussian(varargin)¶
Construct a VSWF representation of a tightly focussed Gaussian beam. Inherits from
ott.beam.BscFinite
andott.beam.properties.Gaussian
.- Properties
waist – Beam waist radius [m]
index_medium – Refractive index of the medium
omega – Optical angular frequency of light [1/s]
position – Position of the beam [m]
rotation – Rotation of the beam [3x3 rotation matrix]
power – Beam power [W]
polbasis – (enum) Polarisation basis (‘polar’ or ‘cartesian’)
polfield – (2 numeric) Field in theta/phi or x/y directions
mapping – Paraxial to far-field beam mapping
data – Internal BSC instance describing beam
- Methods
getData – Get data for specific Nmax
recalculate – Recalculate the beam data
- Static methods
FromNa – Construct a beam specifying NA instead of waist
LaguerreGaussian¶
- class ott.beam.LaguerreGaussian(varargin)¶
Construct a VSWF representation of tightly focussed Laguerre-Gaussian beam. Inherits from
ott.beam.BscFinite
andott.beam.properties.LaguerreGaussian
.- Properties
waist – Beam waist radius [m]
index_medium – Refractive index of the medium
omega – Optical angular frequency of light [1/s]
position – Position of the beam [m]
rotation – Rotation of the beam [3x3 rotation matrix]
power – Beam power [W]
lmode – Azimuthal mode number
pmode – Radial mode number
polbasis – (enum) Polarisation basis (‘polar’ or ‘cartesian’)
polfield – (2 numeric) Field in theta/phi or x/y directions
mapping – Paraxial to far-field beam mapping
data – Internal BSC instance describing beam
- Methods
getData – Get data for specific Nmax
recalculate – Recalculate the beam data
- Static methods
FromNa – Construct a beam specifying NA instead of waist
HermiteGaussian¶
- class ott.beam.HermiteGaussian(varargin)¶
Construct a VSWF representation of tightly focussed Hermite-Gaussian beam. Inherits from
ott.beam.BscFinite
andott.beam.properties.HermiteGaussian
.- Properties
waist – Beam waist radius [m]
index_medium – Refractive index of the medium
omega – Optical angular frequency of light [1/s]
position – Position of the beam [m]
rotation – Rotation of the beam [3x3 rotation matrix]
power – Beam power [W]
mmode – Hermite mode number
nmode – Hermite mode number
polbasis – (enum) Polarisation basis (‘polar’ or ‘cartesian’)
polfield – (2 numeric) Field in theta/phi or x/y directions
mapping – Paraxial to far-field beam mapping
data – Internal BSC instance describing beam
- Methods
getData – Get data for specific Nmax
recalculate – Recalculate the beam data
- Static methods
FromNa – Construct a beam specifying NA instead of waist
InceGaussian¶
- class ott.beam.InceGaussian(varargin)¶
Construct a VSWF representation of tightly focussed Laguerre-Gaussian beam. Inherits from
ott.beam.BscFinite
andott.beam.properties.InceGaussian
.- Properties
waist – Beam waist radius [m]
index_medium – Refractive index of the medium
omega – Optical angular frequency of light [1/s]
position – Position of the beam [m]
rotation – Rotation of the beam [3x3 rotation matrix]
power – Beam power [W]
lmode – Azimuthal mode number
porder – Paraxial mode order.
ellipticity – Ellipticity of coordinates
parity – Parity of beam (‘even’ or ‘odd’)
polbasis – (enum) Polarisation basis (‘polar’ or ‘cartesian’)
polfield – (2 numeric) Field in theta/phi or x/y directions
mapping – Paraxial to far-field beam mapping
data – Internal BSC instance describing beam
- Methods
getData – Get data for specific Nmax
recalculate – Recalculate the beam data
- Static methods
FromNa – Construct a beam specifying NA instead of waist
PlaneWave¶
- class ott.beam.PlaneWave(varargin)¶
VSWF representation of a Plane Wave beam. Inherits from
ott.beam.BscInfinite
.Plane waves support smart translations, where the beam components are phase shifted rather than re-calculated.
- Properties
polarisation – (2 numeric) Polarisation in the x/y directions
Nmax – Nmax of the stored data
data – Internal BSC instance describing beam
Mathieu¶
- class ott.beam.Mathieu(varargin)¶
Construct a VSWF representation of a Mathieu beam. Inherits from
ott.beam.BscInfinite
andott.beam.properties.Mathieu
andott.beam.mixin.BesselBscCast
.- Properties
theta – Annular angle [radians]
morder – Mathieu beam mode number
ellipticity – Ellipticity of Mathieu beam
parity – Parity of beam (‘even’ or ‘odd’)
Webber¶
- class ott.beam.Webber(varargin)¶
Construct a VSWF representation of a Bessel beam. Inherits from
ott.beam.BscInfinite
andott.beam.properties.Webber
.- Properties
theta – Annular angle [radians]
alpha – Parameter describe Webber beam
parity – Parity of beam (either ‘even’ or ‘odd’)
data – Internal BSC instance describing beam
Bessel¶
- class ott.beam.Bessel(varargin)¶
Construct a VSWF representation of a Bessel beam. Inherits from
ott.beam.BscInfinite
andott.beam.properties.Bessel
.See base classes for list of properties/methods.
Annular¶
- class ott.beam.Annular(varargin)¶
Construct a VSWF representation of a finite Annular beam Inherits from
ott.beam.BscWBessel
andott.beam.properties.Polarisation
andott.beam.properties.Profile
andott.beam.properties.Lmode
.Represents beams internally as an array of Bessel-like beams. Applies the beam weights when the data is requested.
- Properties
polbasis – Polarisation basis (‘polar’ or ‘cartesian’)
polfield – Polarisation field (theta/phi or x/y)
theta – Low and upper angles of the annular
profile – Anular profile (function_handle)
lmode – Orbital angular momentum number
- Static methods
InterpProfile – Generate a beam profile using interpolation
BeamProfile – Generate a beam profile from another beam
PmParaxial¶
- class ott.beam.PmParaxial(varargin)¶
Construct a beam using paraxial far-field point matching. Inherits from
BscBeam
andproperties.Mapping
,properties.Polarisation
andproperties.Profile
.- Properties
mapping – Paraxial to far-field mapping
polbasis – (enum) Polarisation basis (‘polar’ or ‘cartesian’)
polfield – (2 numeric) Field in theta/phi or x/y directions
truncation_angle – Maximum angle for beam. Default: []
data – Internal BSC data
- Methods
recalculate – Update the internal data for new Nmax
- Static methods
InterpProfile – Generate a beam profile using interpolation
BeamProfile – Construct beam profile from another beam
- Supported casts
ott.bsc.Bsc – Construct bsc instance
Array types¶
Coherent¶
- ott.beam.Coherent¶
Incoherent¶
- ott.beam.Incoherent¶