drag Package¶
The drag package provides methods for calculating Stokes drag for spherical and non-spherical particles in unbounded free fluid and methods to calculate the wall effects for spheres near walls and eccentric spheres. These methods assume Stokes flow (creeping flow) (i.e., low Reynolds number).
All drag methods inherit from Stokes
. The simplest way to
construct the drag tensor for a set of shapes is to call the static
method Stokes.FromShape()
with the geometry, for example:
shape = ott.shapes.Sphere();
drag = ott.drag.Stokes.FromShape(shape);
Stokes.FromShape()
can also be called with arrays of shapes.
In this case, the method will attempt to generate an array of drag
tensors for the specified configuration and raise a warning or error
when the configuration is unlikely to behave as expected.
Classes in this package can be classified into two main categories: methods for Free particles and methods for Wall effects. A summary of these classes is shown graphically in Fig. 10.
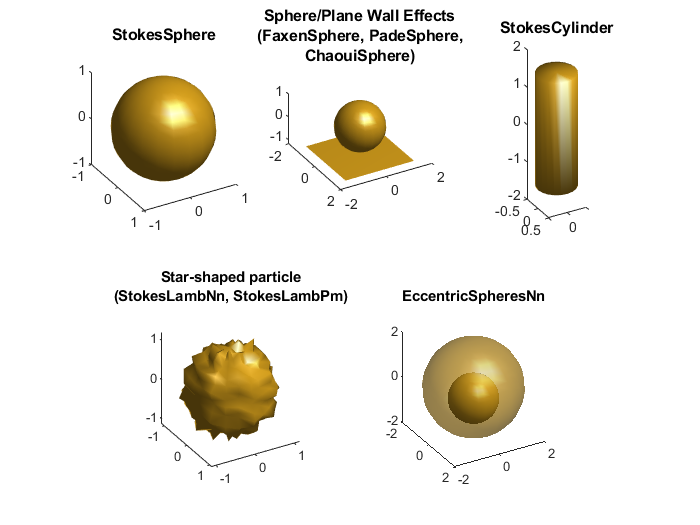
Fig. 10 Graphical display of different particle shapes/scenarious for which drag methods are provided. Other shapes/geometries can be approximated by choosing one of the above drag methods.¶
Contents
Generic classes¶
The base class for all drag tensors is the Stokes
class.
This class specifies the common functionality for drag tensors and
provides the static method for creating drag tensors from shape arrays.
The StokesData
class is an instance of the Stokes
class
which provides data storage of the drag and inverse drag tensors.
Unlike other classes, which compute the drag tensors as needed, the
StokesData
class stores the drag tensors.
All other classes can be cast to the StokesData
class using:
dragData = ott.drag.StokesData(drag);
This operation performs all drag calculations and applies any rotations to the particle; this may provide a performance improvement if the same drag is needed for repeated calculations.
Stokes (base class)¶
- class ott.drag.Stokes(varargin)¶
Base class for 6-vector force/torque drag tensors. Inherits from
ott.utils.RotateHelper
.This class is the base class for drag tensors which can be described by a 3x3 translational, rotational, and cross-term matrices in Cartesian coordinates.
- Properties
forward – Rank 2 forward tensor
inverse – Rank 2 inverse tensor
rotation – Rotation matrix to apply to forward/inverse
- Abstract properties
forwardInternal – Forward tensor without rotation
inverseInternal – Inverse tensor without rotation
- Dependent properties
gamma – Translational component of tensor
delta – Rotational component of tensor
crossterms – Cross-terms component of tensor (UD)
igamma – Inverse translational component of tensor
idelta – Inverse rotational component of tensor
icrossterms – Inverse cross-terms component of tensor (UD)
- Methods
inv – Return the inverse or calculate the inverse
mtimes – Multiply the tensor or calculate the forward and mul
vecnorm – Compute vecnorm of tensor rows
diag – Get the diagonal of the forward tensor
rotate* – Rotate the tensor around the X,Y,Z axis
- Static methods
FromShape – Construct a drag tensor from a shape or array
- Supported casts
double – Get the forward drag tensor
StokesData – Pre-computes forward/inverse tensors
ott.shape.Shape – Gets a geometrical representation of the object
See also
StokesSphere
andStokesLambNn
.- static FromShape(shape, varargin)¶
Attempt to select an appropriate drag calculation method.
Not all shapes are supported, most shapes default to a sphere whose radius matches the maxRadius of the shape.
If the input is a single shape, attempts to choose an appropriate method for the shape. May raise warnings if no good method is found.
If the input contains a plane and a finite extent particle, models the particle as a sphere near a wall. Raises a warning if the particle is not a sphere.
If the input contains a particle inside the circumscribing sphere of another particle, uses EccentricSpheresNn. Raises a warning if the particles are not spheres.
For all other arrays, assumes the particles are non-interacting and generates drag tensors for each particle. If the particles are within 5 radii of each other, raises a warning.
- Usage
drag = Stokes.FromShape(shape, eta, …)
- Parameters
shape (ott.shape.Shape) – A shape or array of shapes.
eta (numeric) – Viscosity (passed to class constructor).
Additional parameters passed to class constructors.
- Stokes(varargin)¶
Construct a new drag tensor instance.
- Usage
drag = Stokes(…)
- Optional named parameters
rotation (3x3 numeric) – Initial rotation property. Default:
eye(3)
.
StokesData¶
- class ott.drag.StokesData(varargin)¶
Stokes drag tensor definition with explicit forward and inverse data
- Properties
inverse – (6x6 numeric) inverse drag tensor
forward – (6x6 numeric) forward drag tensor
Additional methods/properties inherited from
Stokes
.- StokesData(varargin)¶
Construct new Stokes drag tensor instance with explicit data
- Usage
drag = StokesDrag(forward, inverse, …)
- Parameters
forward (6x6 numeric) – Forward drag tensor
inverse (6x6 numeric) – Inverse drag tensor
If either of forward or inverse are omitted, they are calculated from the corresponding tensor.
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
Free particles¶
These classes calculate drag tensors for particles in unbounded free fluid.
Spheres have an analytical solution and can be calculated using
StokesSphere
.
Arbitrary shapes can be calculated in spherical
coordinates using point-matching using Lamb Series via StokesLambPm
.
StokesLambNn
is a pre-trained neural network for the Lamb
series solution for arbitrary shaped particles which enables faster
drag prediction with comparable accuracy to StokesLambPm
.
For Cylinders or elongated particles with high aspect ratios it may
be better to use the StokesCylinder
approximation instead.
StokesSphere¶
- class ott.drag.StokesSphere(varargin)¶
Drag tensor for a sphere with Stokes Drag
- Properties
radius – Radius of sphere
viscosity – Viscosity of medium
forward – Calculated drag tensor
inverse – Calculate from forward
- Supported casts
ott.shape.Shape – Constructs a sphere shape
See
Stokes
for other methods/parameters.- StokesSphere(varargin)¶
Calculate drag tensors for spherical particle in Stokes drag.
- Usage:
tensor = Sphere(radius, viscosity, …)
- Parameters
radius – (numeric) Radius of particle (default: 1.0)
viscosity – (numeric) Viscosity of medium (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
StokesCylinder¶
- class ott.drag.StokesCylinder(varargin)¶
Drag tensor for long/slender cylindrical particles.
Uses the results from
Maria M. Tirado and José García de la Torre J. Chem. Phys. 71, 2581 (1979); https://doi.org/10.1063/1.438613
And from
María M. Tirado and José García de la Torre J. Chem. Phys. 73, 1986 (1980); https://doi.org/10.1063/1.440288
which provide lookup tables for the drag corrections for slender cylinders (aspect ratio height/diameter above 2).
- Properties
radius – Cylinder radius
height – Cylinder height
viscosity – Medium viscosity
forward – Calculated drag tensor
inverse – Inverse drag tensor (calculated from forward)
- Supported casts
ott.shape.Shape – Construct a cylinder
Additional properties/methods inherited from
Stokes
.- StokesCylinder(varargin)¶
Calculate drag tensors for cylindrical particles
- Usage:
tensor = Sphere(radius, height, viscosity, …)
- Parameters
radius – (numeric) Radius of cylinder (default: 1.0)
height – (numeric) Height of cylinder (default: 4.0)
viscosity – (numeric) Viscosity of medium (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
StokesLambNn¶
- class ott.drag.StokesLambNn(varargin)¶
Calculate stokes drag for star shaped particles using pre-trained NN. Inherits from
StokesStarShaped
.Uses the neural network from Lachlan Gibson’s PhD thesis (2016).
- Properties
inverse – Calculated from forward
forward – Drag tensor calculated using point matching.
viscosity – Viscosity of medium
shape – A star shaped particle describing the geometry
- Static methods
SphereGrid – Generate grid of angular points for NN
LoadNetwork – Load the neural network
Additional methods/properties inherited from
Stokes
.- StokesLambNn(varargin)¶
Construct star-shaped drag method using Neural network approach.
- Usage
drag = StokesLambNn(shape, …)
- Parameters
shape (ott.shape.Shape) – Star shaped particle.
Parameters can also be passed as named arguments. Additional parameters passed to base.
StokesLambPm¶
- class ott.drag.StokesLambPm(varargin)¶
Calculate drag coefficients using Lamb series and point matching. Inherits from
StokesStarShaped
- Properties
inverse – Calculated from forward
forward – Drag tensor calculated using point matching.
viscosity – Viscosity of medium
shape – A star shaped particle describing the geometry
Additional methods/properties inherited from
Stokes
.Based on and incorporating code by Lachlan Gibson (2016)
- StokesLambPm(varargin)¶
Construct star-shaped drag method using point-matching method.
- Usage
drag = StokesLambPm(shape, …)
- Parameters
shape (ott.shape.Shape) – Star shaped particle.
Parameters can also be passed as named arguments. Additional parameters passed to base.
Wall effects¶
The toolbox includes several approximation methods for calculating
drag for spheres near walls.
The EccentricSpheresNn
can be used to simulate a sphere inside
another sphere or by making the outer sphere radius significantly larger
than the inner sphere, a sphere near a wall.
FaxenSphere
, ChaouiSphere
and PadeSphere
provide drag tensors for spheres near walls with varying approximations.
FaxenSphere
may provide slightly faster calculation time
compared to the other methods but only works well when the particle
is a fair distance from the wall.
EccentricSpheresNn¶
- class ott.drag.EccentricSpheresNn(varargin)¶
Calculate drag on an eccentric sphere using Gibson’s NN approach. Inherits from
Stokes
.Uses the NN from
Lachlan J. Gibson, et al. Phys. Rev. E 99, 043304 https://doi.org/10.1103/PhysRevE.99.043304
- Properties
innerRadius – Radius of inner sphere
outerRadius – Radius of outer sphere
separation – Minimum separation between spheres
viscosity – Viscosity of surrounding fluid (default: 1.0)
forward – Computed drag tensor
inverse – Computed from forward.
See
Stokes
for other methods/parameters.
FaxenSphere¶
- class ott.drag.FaxenSphere(varargin)¶
Stokes drag with Faxen’s corrections for movement near a plane. Inherits from
StokesSphereWall
.Faxen’s correction can provide reasonable estimates for the drag on a spherical particle moving near a planar surface. The approximation works well to within about 1 particle radius from the surface.
This implementation uses the Faxen’s corrections described in
J. Leach, et al. Phys. Rev. E 79, 026301 https://doi.org/10.1103/PhysRevE.79.026301
For the rotational-translational Faxen’s coupling, we use Eq. 7-4.29 from
John Happel and Howard Brenner, Low Reynolds number hydrodynamics, (1983) https://doi.org/10.1007/978-94-009-8352-6
This class assumes the surface is perpendicular to the z axis, positioned bellow the spherical particle.
- Properties
radius – Radius of the sphere
viscosity – Viscosity of the medium
separation – Distance between the sphere centre and plane
forward – Computed drag tensor
inverse – Computed from forward.
See
Stokes
for other methods/parameters.- FaxenSphere(varargin)¶
Construct a new Faxen’s corrected sphere drag tensor.
- Usage
drag = FaxenSphere(radius, separation, viscosity, …) Radius and separation should be specified in the same units.
- Parameters
radius – (numeric) Radius of sphere (default: 1)
separation – Separation between sphere centre and surface
viscosity – Viscosity of medium (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
PadeSphere¶
- class ott.drag.PadeSphere(varargin)¶
Creeping flow around a sphere in shear flow near to a wall. Inherits from
StokesSphereWall
.This class implements the Pade series approximation for spherical particles moving near a planar surface. The approximation should work for spacing between the sphere surface and plane larger than \(10^{-2}\) radius.
This class uses the coefficients included in
M. Chaoui and F. Feuillebois, Creeping Flow Around a Sphere in a Shear Flow Close to a Wall. The Quarterly Journal of Mechanics and Applied Mathematics, Volume 56, Issue 3, August 2003, Pages 381–410, https://doi.org/10.1093/qjmam/56.3.381
This class assumes the surface is perpendicular to the z axis, positioned bellow the spherical particle.
- Properties
radius – Radius of the sphere
viscosity – Viscosity of the medium
separation – Distance between the sphere centre and plane
forward – Computed drag tensor
inverse – Computed from forward.
See
Stokes
for other methods/parameters.- PadeSphere(varargin)¶
Construct a new creeping flow sphere-wall drag tensor.
- Usage:
drag = PadeSphere(radius, separation, viscosity, …) radius and separation should be specified in the same units.
- Parameters:
radius – Radius of sphere (default: 1.0)
separation – Separation between sphere centre and surface
viscosity – Viscosity of medium (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
ChaouiSphere¶
- class ott.drag.ChaouiSphere(varargin)¶
Creeping flow around a sphere close to a wall. Inherits from
StokesSphereWall
.This class implements a polynomial fit to the exact solution for spherical particles moving in creeping flow near a planar surface. The approximation should work for spacing between the sphere surface and plane between \(10^{-6}\) radius and 1 radius.
This class implements the polynomial approximation described in
M. Chaoui and F. Feuillebois, Creeping Flow Around a Sphere in a Shear Flow Close to a Wall. The Quarterly Journal of Mechanics and Applied Mathematics, Volume 56, Issue 3, August 2003, Pages 381–410, https://doi.org/10.1093/qjmam/56.3.381
This class assumes the surface is perpendicular to the z axis, positioned bellow the spherical particle.
- Properties
radius – Radius of the sphere
viscosity – Viscosity of the medium
separation – Distance between the sphere centre and plane
forward – Computed drag tensor
inverse – Computed from forward.
See
Stokes
for other methods/parameters.- ChaouiSphere(varargin)¶
Construct a new creeping flow sphere-wall drag tensor.
- Usage:
drag = ChaouiSphere(radius, separation, viscosity, …) radius and separation should be specified in the same units.
- Parameters:
radius – Radius of sphere
separation – Separation between sphere centre and surface
viscosity – Viscosity of medium (optional, default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
Abstract base classes¶
In addition to the Stokes
abstract base class, the following
classes can also be used for implementing custom drag classes.
StokesSphereWall¶
- class ott.drag.StokesSphereWall(varargin)¶
Abstract base class for sphere-wall drag calculation methods. Inherits from
Stokes
.- Properties
radius – Radius of sphere
separation – Distance from sphere centre to wall
viscosity – Viscosity of medium
inverse – Calculated from forward
- Abstract properties
forward – Drag tensor calculated by method
- Static methods
FromShape – Construct a drag tensor from a shape array
- Supported casts
ott.shape.Shape – Cast to plane and sphere
- static FromShape(shape, varargin)¶
Attempt to choose a sphere-wall drag method based on a shape array
- Usage
drag = StokesSphereWall.FromShape(shape, …)
- Parameters
shape (ott.shape.Shape) – Array of shapes. Must be two elements, one of which must be a plane.
Additional parameters are passed to corresponding class constructor.
- StokesSphereWall(varargin)¶
Construct base class for sphere-wall methods
- Usage
drag = drag@ott.drag.StokesSphereWall(radius, separation, viscosity, …)
- Parameters
radius (numeric) – Particle radius (default: 1.0)
separation (numeric) – Separation (default: Inf)
viscosity (numeric) – Viscosity (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.
StokesStarShaped¶
- class ott.drag.StokesStarShaped(varargin)¶
Abstract base class for star shaped particle methods in an unbounded medium. Inherits from
Stokes
.- Properties
inverse – Calculated from forward
viscosity – Viscosity of medium
shape – A star shaped particle describing the geometry
- Abstract properties
forwardInternal – Drag tensor calculated by method
- Static methods
FromShape – Defers to StokesLambNn (may change in future)
- Supported casts
ott.shape.Shape – Retrieves the geometry (see shape)
- static FromShape(shape, varargin)¶
Construct drag tensor for star shaped particle
- Usage
StokesStarShaped.FromShape(shape, …)
Passes all arguments to
StokesLambNn
.
- StokesStarShaped(varargin)¶
Construct base class for star-shaped particle methods
- Usage
drag = drag@ott.drag.StokesStarShaped(shape, viscosity, …)
- Parameters
shape (ott.shape.Shape) – A shape object with a starShaped property with the true value.
viscosity (numeric) – Viscosity of medium (default: 1.0)
Parameters can also be passed as named arguments. Unmatched parameters are passed to
Stokes
.